Giới thiệu
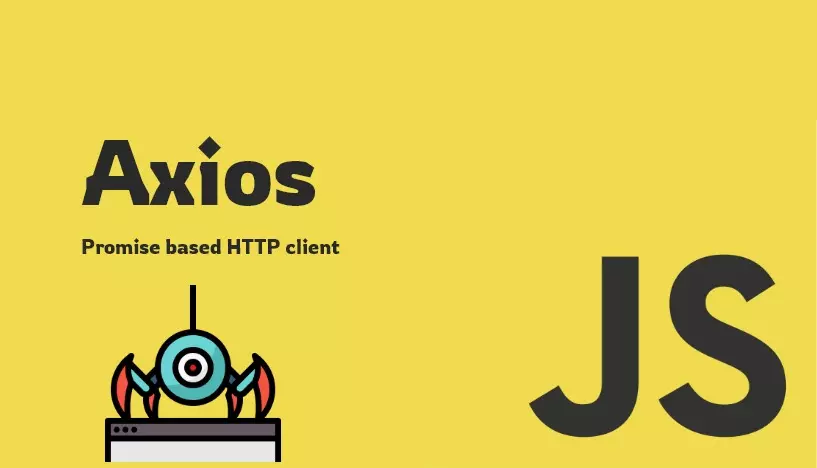
Axios là gì?
Axios là một HTTP client dựa được phát triển trên đối tượng Javascript Promise, nó có thể sử dụng trong các ứng dụng font-end Vue.js, React, Angular… Sử dụng Axios dễ dàng gửi đi các request HTTP bất đồng bộ đến các REST endpoint và thực hiện các chức năng CRUD. Chúng ta gặp phải một số khái niệm có thể nhiều bạn chưa biết đến:
HTTP client là có thể là phần mềm, thư viện có thể thực hiện các yêu cầu (request) dạng HTTP đến máy chủ HTTP và nhận về các hồi đáp (reponse). Đơn giản hơn bạn có thể coi nó gần với một trình duyệt web.
Javascript Promise là một đối tượng giúp kiểm soát kết quả hoàn thành hay thất bại của một hành động bất đồng bộ trong Javascript (Tham khảo thêm Kiến thức về Javascript Promise). Vue.js, React, Angular là những framework Javascript hiện đang rất hot, giúp xây dựng những ứng dụng font-end linh hoạt hoạt động nhanh và mạnh mẽ.
REST endpoint là những điểm (URL) cung cấp các chức năng API cho phép chúng ta tương tác với một hệ thống, ví dụ khi chúng ta muốn tương tác với Lazada chúng ta có thể gửi các request HTTP đến các REST API do Lazada cung cấp.
CRUD viết tắt của Create, Read, Update, Delete là một thuật ngữ lập trình nói đến 4 phương thức quen thuộc khi làm việc với kho dữ liệu.
Cài đặt
reactjs
npx create-react-app learn-reactjs
npminstall --save react-router-dom
cd learn-reactjs
npm start
axios
npm i --save axios
Tạo các thư mục
learn-reactjs
├─ build
├─ node_modules
├─ public
└─ src
├─ api
│ ├─ axiosClient.js
│ └─ productApi.js
├─ app
│ └─ store.js
├─ components
├─ features
│ └─ Product
│ ├─ components
│ │ ├─ Product.jsx
│ │ └─ ProductList.jsx
│ └─ pages
│ │ └─ ListPage.jsx
│ └─ index.jsx
├─ App.css
├─ App.js
└─ index.js
App
Chỉnh sửa file index.js
import React from'react';import ReactDOM from'react-dom';import{ BrowserRouter }from'react-router-dom';import App from'./App';import'./index.css';import reportWebVitals from'./reportWebVitals';
ReactDOM.render(<React.StrictMode><BrowserRouter><App /></BrowserRouter></React.StrictMode>,
document.getElementById('root'));// If you want to start measuring performance in your app, pass a function// to log results (for example: reportWebVitals(console.log))// or send to an analytics endpoint. Learn more: https://bit.ly/CRA-vitalsreportWebVitals();
Chỉnh sửa file app.js
import{ Redirect, Route, Switch }from'react-router-dom';import'./App.css';import ProductFeature from'./features/Product';functionApp(){return(<div className="app"><Switch><Redirect from="home" to="/" exact /><Route path="/products" component={ProductFeature}/></Switch></div>);}exportdefault App;
Api
axiosClient
Bạn tạo một phiên bản axios mới với cấu hình tùy chỉnh bằng cách chỉnh sửa file axiosClient.js trong thư mục api.
import axios from'axios';const axiosClient = axios.create({
baseURL:'http://127.0.0.1:8000/',
headers:{'content-type':'application/json',}})
productApi
Chỉnh sửa file productApi.js. File này có mục đích dùng để lấy dữ liệu products.
import axiosClient from"./axiosClient";const productApi ={asyncgetAll(params){var qs =require('qs');const response =await axiosClient.get('products/',{
params:{...params,},paramsSerializer:(params)=>{//ví dụ với trường hợp size=[1,2] => &size=1&size=2return qs.stringify(params,{ arrayFormat:'repeat'})},})return response
},get(id){const url =`/products/${id}/`;return axiosClient.get(url);},add(data){const url =`/products/`;return axiosClient.post(url, data);},update(data){const url =`/products/${data.id}/`;return axiosClient.patch(url, data);},remove(id){const url =`/products/${id}/`;return axiosClient.delete(url);},}exportdefault productApi
Product
Chỉnh sửa file index.jsx trong thư mục product
import React from'react';import{ Route, Switch, useRouteMatch }from'react-router-dom';import ListPage from'./pages/ListPage';
ProductFeature.propTypes ={};functionProductFeature(props){const match =useRouteMatch()return(<div><Switch><Route path={match.url} exact><ListPage/></Route></Switch></div>);}exportdefault ProductFeature;
List Page
Tiếp đến chỉnh sửa file ListPage.jsx trong thư mục Product/Page
import React,{ useEffect, useState }from'react';import productApi from'../../../api/productApi';import ListProduct from'../components/views/ProductList';functionListPage(props){const[products, setProducts]=useState([])const[loading, setLoading]=useState(true)const[filters, setFilters]=useState({});useEffect(()=>{;(async()=>{try{const response =await productApi.getAll(filters)setProducts(response.data.results)}catch(error){
console.log(error.message)}setLoading(false)})()},[filters])// nếu bạn muốn tìm kiếm thì hãy gọi hàm này để thay đổi fillterconstfilterChange=(newFilters)=>{setLoading(true)setFilters(newFilters)}return(<div><ProductList products={products}/></div>);}exportdefault ListPage;
Product List
Chỉnh sửa file ProductList.jsx trong thư mục Product/components
import PropTypes from'prop-types';import React from'react';import Product from'./Product';
ProductList.propTypes ={
products: PropTypes.array,};
ProductList.defaultProps ={
products:[],}functionProductList(props){const{ products}= props
return(<div><ul>{products.map(product=>(<li><Product product={product}/></li>)}<ul></div>);}exportdefault ProductList;
Product
Chỉnh sửa file product.jsx trong thư mục Product/component
import PropTypes from'prop-types';import React,{ useState }from'react';
Product.propTypes ={
product: PropTypes.object,};
Product.defaultProps ={
product:{},}functionProduct(props){const{ product }= props
return(<div>{product.title}</div>);}exportdefault Product;
Bài viết đến đây là kết thúc. Chúc các bạn thành công
Nguồn: viblo.asia